- Back to Home »
- ADO.NET Entity Framework , LINQ »
- LINQ TUTORIAL PART 5 - LINQ to ENTITIES
Posted by :
Sudhir Chekuri
Wednesday, 28 October 2015
LINQ to Entities
Construct ObjectQuery instance out of ObjectContext.
Create a query in C#.NET
Conversion of Linq operators and expressions into command trees.
Executing query with exceptions
Returning all the results to client directly.
ObjectContext used by Linq queries to connect to database.
Step by step example for linq to entities in Console applications:
In this example data from SQL Server database table is displayed, inserted, updated and deleted from console application
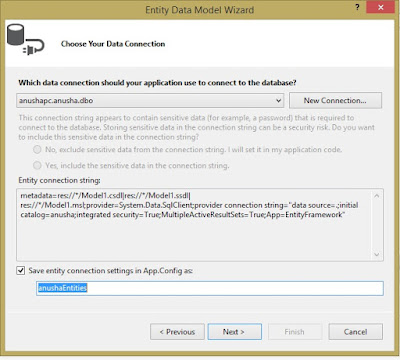
Construct ObjectQuery instance out of ObjectContext.
Create a query in C#.NET
Conversion of Linq operators and expressions into command trees.
Executing query with exceptions
Returning all the results to client directly.
ObjectContext used by Linq queries to connect to database.
Step by step example for linq to entities in Console applications:
In this example data from SQL Server database table is displayed, inserted, updated and deleted from console application
- Create database table using below query and insert some data
create table tbl_student(sno int identity primary key, name varchar(20),course varchar(20))
insert into tbl_student values('sudhir','abcd') - Create a console application
Right click on project name in solution explorer and click on add item
Select ADO,NET ENTITY DATA MODEL.
- Click on Add
- Select generate from database and click on Next
- Click on Add new connection to create a new connection the database server
- Enter server name, select authentication, select database in which you created the database
- Click on test connection to check the connection. If it is passed then click on OK.
- Select the save entity connection settings check box and click on NEXT.
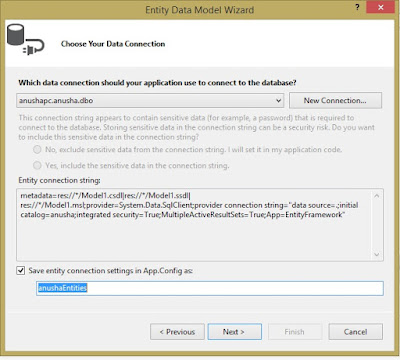
- Select the table and remember the Model namespace name which we use in the code
- Click on Finish.
Example program in console application for CRUD operations with LINQ to Entities
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LinqApp
{
class Program
{
static void Main(string[] args)
{
using (anushaEntities context = new anushaEntities())
{
//Get the List of student details from Student Database table
var studentList = from s in context.tbl_student
select s;
//Display the List of student details from Student Database table
//Use this loop after every operation to see the updated data from the database
//studentList contains table data and row by row is retrieved to stud to display all details
foreach (var stud in studentList)
{//name and course from every row is displayed
Console.WriteLine("Student Name " + stud.name + ", Course " + stud.course);
}
//Add new student details
//Created new student details using an object
tbl_student student = new tbl_student() { name = "Raj", course = "PHP" };
//Adding details using student object to the table
context.tbl_student.Add(student);
//Saving data to database using db context
context.SaveChanges();
//Printing success message to user
Console.WriteLine("Student details is inserted in Database");
////Update existing Student details
//First row in student table matched with condition is returned
var studUpdate = context.tbl_student.FirstOrDefault(s => s.name == "sudhir");
//Course value in returned row is updated with new value
studUpdate.course = "Azure";
//Updated changes are saved to database
context.SaveChanges();
//Success message is displayed to user
Console.WriteLine("Student details updated in Database");
////Delete existing student details
////First row in student table matched with condition is returned
var studDelete = context.tbl_student.FirstOrDefault(s => s.name == "sudhir");
////Returned row is deleted using context table
context.tbl_student.Remove(studDelete);
//Changes are saved to database
context.SaveChanges();
//Success message is displayed to user
Console.WriteLine("Student details deleted in Database");
}
Console.WriteLine("\nPress any key to continue.");
Console.ReadKey();
}
}
}