Archive for October 2015
Microsoft Azure Part - 2 How Websites Works?
Web hosting plans:
One azure subscription can have one or more website plans.One azure subscription can have one or more resource groups.
A resource group can have virtual machines, websites,...
Azure websites deployed into the same subscription, resource group and geographic location can share a single web hosting plan.
Each Azure Website sharing a web hosting plan can leverage all the capabilities of that plan.
Each Azure Website sharing a web hosting plan can leverage all the capabilities of that plan.
In azure portal by selecting websites from left pane - Click on website - Under scale tab - you can select web hosting plan mode.
Link for : How Azure pricing works?
From the second time it has information in cache about where the application is located i.e., in which web worker.
Hot website: One website is hosted in a physical machine in one web worker. If one web worker goes down it you cannot get response for your application.
If your website is hosted in more than one physical machine(Web workers). Then if one web worker goes down the request is redirected to other web worker which will grab resources from other physical locations available.
Scaling: With shared plan your website is deployed to two different servers(Instances) and the requested are shared among the instances which is known as scaling.
Scaling can be done by Scaling Up or Scaling Out.
Scaling up: Increasing processing power or resources of an instance is known as scaling up.
Scaling out: Increasing number of instances or servers is known as Scaling out. This helps in sharing the request coming in for the application by different servers.
There are two types of instances:
1. Shared Instances
- Free plan
- Shared plan
2. Reserved Instances
- Basic Plan
- Standard Plan
In Reserved Instances(Basic and Standard)
In Reserved Instances IIS will be in Always on mode i.e., it is always in active state and waiting for giving immediate response for the request. By default, IIS will not be active for more than 20 minutes there is no request coming to IIS.
In shared plan you are scaling out to multiple dedicated compute instances in which other websites are not hosted other than your application.
SSL certificates, Web sockets and SLA 99.9% features are available.
In Standard plan
Scale out to up to 10 dedicated compute instances.
Bacups, Autoscale, Azure Scheduler, Staged Publishing is available.
Scaling up can be done by creating small, medium, large instances also by increasing, RAM and CPU Cores.
How website works?
When first time the application(Website) is request it doesn't have information about the location of the application. Then request is delegated to the web worker. Then web worker will grab information from all locations like hosting sites DB, storage controller and site's content DB and gives response back to the IIS Application Request Router and then web page is given back to the user.From the second time it has information in cache about where the application is located i.e., in which web worker.
Hot website: One website is hosted in a physical machine in one web worker. If one web worker goes down it you cannot get response for your application.
If your website is hosted in more than one physical machine(Web workers). Then if one web worker goes down the request is redirected to other web worker which will grab resources from other physical locations available.
Scaling can be done by Scaling Up or Scaling Out.
Scaling up: Increasing processing power or resources of an instance is known as scaling up.
Scaling out: Increasing number of instances or servers is known as Scaling out. This helps in sharing the request coming in for the application by different servers.
There are two types of instances:
1. Shared Instances
- Free plan
- Shared plan
2. Reserved Instances
- Basic Plan
- Standard Plan
In Reserved Instances(Basic and Standard)
In Reserved Instances IIS will be in Always on mode i.e., it is always in active state and waiting for giving immediate response for the request. By default, IIS will not be active for more than 20 minutes there is no request coming to IIS.
In shared plan you are scaling out to multiple dedicated compute instances in which other websites are not hosted other than your application.
SSL certificates, Web sockets and SLA 99.9% features are available.
In Standard plan
Scale out to up to 10 dedicated compute instances.
Bacups, Autoscale, Azure Scheduler, Staged Publishing is available.
Scaling up can be done by creating small, medium, large instances also by increasing, RAM and CPU Cores.
Microsoft Azure Part - 1 Website Creation and hosting/publishing using Visual Studio
You should have a Microsoft Azure account to work on Azure which is a Microsoft cloud platform. It helps you to host your applications, databases and lot more in cloud which can be managed easily using azure management portal.
Azure portal link
https://manage.windowsazure.com/
You can create a 30 day trial account by signing up using credit card details.
1 $ will be charged for account creation and you can use the cloud services upto $200 for free within 30 days.
If you want to use more data you have to accept pay as you use option. Amount will be charged from your credit card after you accept it.
Usage details will be available in the portal.
Open visual studio 2013 or later versions(I am using VS 2015 community for this example).
Create a new project - Web - ASP.NET Web application using .NET Framework 4.5.
Select Empty template - Select a check box host in cloud and select Web app from dropdown under Microsoft Azure block.
Now configure Microsoft Azure Web app
Enter app name and also service, plan names if they are not configured in portal and using Azure account for the first time.
Click on Ok and it will create a website in Azure. You can also see it in the azure portal by going through websites tab in azure portal.
Now add a web form in visual studio and design the page with some content like hello world.
Check the output in the local host in visual studio.
Hosting it to azure:
Right click on the project root folder and click on publish.
Give some options required to validate connection and selecting publish method as Web Deploy.
Click on Next to select release - Next
Have a preview of the files that you are publishing click on publish to publish it to azure cloud.
Now after successful publishing our website in azure will get launched automatically in the browser. and refresh the page to see the output of your site.
Snapshot of azure portal after publishing website to azure.
Configuring Startup page for azure site:
Open azure portal - Click on Web apps in left pane - Click on the web site name
Go to configure tab - Go to default documents section where you find existing default page names. You can also add new or remove existing default document names here.
Video tutorial link
Microsoft Virtual Academy Azure Website Link
Authentication:
Authentication is of different types:
1. Using login and password in website.
2. Using management certificates using private key and public key to authenticate services.
A single subscription can have 100 certificates only. So 100 apps can use authentication using certificates.
3. Three roles owner, contributor and viewer
How to get Azure certificate?
Login into Azure management portal and open the below link to download the certificate which you can use to authenticate with certificate. It is an xml file contains key to authenticate to azure from visual studio.
https://manage.windowsazure.com/publishsettings/index
Using Azure certificate in visual studio through import option?
Right click on Azure Root folder in Server explorer and click on manage and filter subscriptions.
Under certificates tab import option can be used to browse and select downloaded certificate from portal and click on import. You can see 1 certificate added under certificates tab.
Make sure that you have added your subscription details as well.
Reference link: Azure certificates
In your web project you can see .pubxml file in properties -> PublishProfiles folder which is used to store publish settings.
In the same folder you will also have your encrypted password saving file but it is only visible if you go to that folder location but you can't see it in solution explorer of visual studio for security reasons.
This password is used to authenticate while publishing files and project from different resources to Azure.
A publishsettings file is also known as an Account file.
Azure portal link
https://manage.windowsazure.com/
You can create a 30 day trial account by signing up using credit card details.
1 $ will be charged for account creation and you can use the cloud services upto $200 for free within 30 days.
If you want to use more data you have to accept pay as you use option. Amount will be charged from your credit card after you accept it.
Usage details will be available in the portal.
Open visual studio 2013 or later versions(I am using VS 2015 community for this example).
Create a new project - Web - ASP.NET Web application using .NET Framework 4.5.
Select Empty template - Select a check box host in cloud and select Web app from dropdown under Microsoft Azure block.
Now configure Microsoft Azure Web app
Enter app name and also service, plan names if they are not configured in portal and using Azure account for the first time.
Click on Ok and it will create a website in Azure. You can also see it in the azure portal by going through websites tab in azure portal.
Now add a web form in visual studio and design the page with some content like hello world.
Check the output in the local host in visual studio.
Hosting it to azure:
Right click on the project root folder and click on publish.
Give some options required to validate connection and selecting publish method as Web Deploy.
Click on Next to select release - Next
Have a preview of the files that you are publishing click on publish to publish it to azure cloud.
Now after successful publishing our website in azure will get launched automatically in the browser. and refresh the page to see the output of your site.
Snapshot of azure portal after publishing website to azure.
Configuring Startup page for azure site:
Open azure portal - Click on Web apps in left pane - Click on the web site name
Go to configure tab - Go to default documents section where you find existing default page names. You can also add new or remove existing default document names here.
Video tutorial link
Microsoft Virtual Academy Azure Website Link
Authentication:
Authentication is of different types:
1. Using login and password in website.
2. Using management certificates using private key and public key to authenticate services.
A single subscription can have 100 certificates only. So 100 apps can use authentication using certificates.
3. Three roles owner, contributor and viewer
How to get Azure certificate?
Login into Azure management portal and open the below link to download the certificate which you can use to authenticate with certificate. It is an xml file contains key to authenticate to azure from visual studio.
https://manage.windowsazure.com/publishsettings/index
Using Azure certificate in visual studio through import option?
Right click on Azure Root folder in Server explorer and click on manage and filter subscriptions.
Under certificates tab import option can be used to browse and select downloaded certificate from portal and click on import. You can see 1 certificate added under certificates tab.
Make sure that you have added your subscription details as well.
Reference link: Azure certificates
In your web project you can see .pubxml file in properties -> PublishProfiles folder which is used to store publish settings.
In the same folder you will also have your encrypted password saving file but it is only visible if you go to that folder location but you can't see it in solution explorer of visual studio for security reasons.
This password is used to authenticate while publishing files and project from different resources to Azure.
A publishsettings file is also known as an Account file.
LINQ TUTORIAL PART 5 - LINQ to ENTITIES
LINQ to Entities
Construct ObjectQuery instance out of ObjectContext.
Create a query in C#.NET
Conversion of Linq operators and expressions into command trees.
Executing query with exceptions
Returning all the results to client directly.
ObjectContext used by Linq queries to connect to database.
Step by step example for linq to entities in Console applications:
In this example data from SQL Server database table is displayed, inserted, updated and deleted from console application
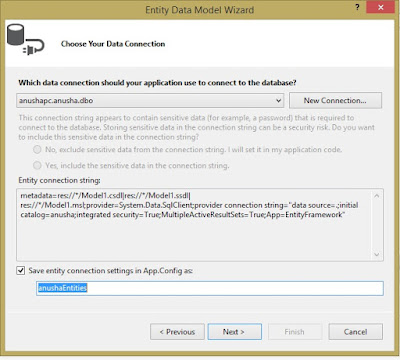
Construct ObjectQuery instance out of ObjectContext.
Create a query in C#.NET
Conversion of Linq operators and expressions into command trees.
Executing query with exceptions
Returning all the results to client directly.
ObjectContext used by Linq queries to connect to database.
Step by step example for linq to entities in Console applications:
In this example data from SQL Server database table is displayed, inserted, updated and deleted from console application
- Create database table using below query and insert some data
create table tbl_student(sno int identity primary key, name varchar(20),course varchar(20))
insert into tbl_student values('sudhir','abcd') - Create a console application
Right click on project name in solution explorer and click on add item
Select ADO,NET ENTITY DATA MODEL.
- Click on Add
- Select generate from database and click on Next
- Click on Add new connection to create a new connection the database server
- Enter server name, select authentication, select database in which you created the database
- Click on test connection to check the connection. If it is passed then click on OK.
- Select the save entity connection settings check box and click on NEXT.
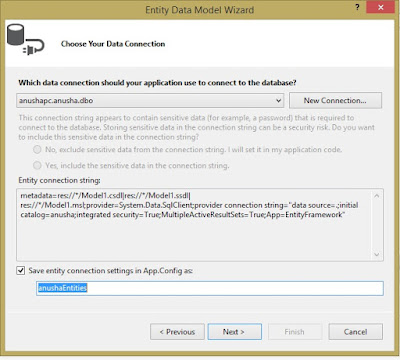
- Select the table and remember the Model namespace name which we use in the code
- Click on Finish.
Example program in console application for CRUD operations with LINQ to Entities
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LinqApp
{
class Program
{
static void Main(string[] args)
{
using (anushaEntities context = new anushaEntities())
{
//Get the List of student details from Student Database table
var studentList = from s in context.tbl_student
select s;
//Display the List of student details from Student Database table
//Use this loop after every operation to see the updated data from the database
//studentList contains table data and row by row is retrieved to stud to display all details
foreach (var stud in studentList)
{//name and course from every row is displayed
Console.WriteLine("Student Name " + stud.name + ", Course " + stud.course);
}
//Add new student details
//Created new student details using an object
tbl_student student = new tbl_student() { name = "Raj", course = "PHP" };
//Adding details using student object to the table
context.tbl_student.Add(student);
//Saving data to database using db context
context.SaveChanges();
//Printing success message to user
Console.WriteLine("Student details is inserted in Database");
////Update existing Student details
//First row in student table matched with condition is returned
var studUpdate = context.tbl_student.FirstOrDefault(s => s.name == "sudhir");
//Course value in returned row is updated with new value
studUpdate.course = "Azure";
//Updated changes are saved to database
context.SaveChanges();
//Success message is displayed to user
Console.WriteLine("Student details updated in Database");
////Delete existing student details
////First row in student table matched with condition is returned
var studDelete = context.tbl_student.FirstOrDefault(s => s.name == "sudhir");
////Returned row is deleted using context table
context.tbl_student.Remove(studDelete);
//Changes are saved to database
context.SaveChanges();
//Success message is displayed to user
Console.WriteLine("Student details deleted in Database");
}
Console.WriteLine("\nPress any key to continue.");
Console.ReadKey();
}
}
}
LINQ TUTORIAL PART 4 - LINQ to XML
LINQ to XML is used to query data from xml document using simple queries so that we can save data from xml in other formats.
Example program for LINQ to XML:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQApp
{
class Program
{
static void Main(string[] args)
{
//xml data in saved in string
string myXML = @"<Students>
<StudentName>Amir</StudentName>
<StudentName>Salman</StudentName>
<StudentName>Sharukh</StudentName>
<StudentName>Hrithik</StudentName>
</Students>";
XDocument xdoc = new XDocument();
//Converting data in string to XDocument format.
xdoc = XDocument.Parse(myXML);
//Quering all elements under Students tag
var result = xdoc.Element("Students").Descendants();
//Printing Values inside all retreived nodes(Nodes inside Students node)
foreach (XElement item in result)
{
Console.WriteLine("Student Name - " + item.Value);
}
Console.ReadKey();
}
}
}
Example program to Add new xml tags in exiting xml document:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQApp
{
class Program
{
static void Main(string[] args)
{
string myXML = @"<Courses>
<Course>PHP</Course>
<Course>JAVA</Course>
<Course>DOT NET</Course>
</Courses>";
XDocument xdoc = new XDocument();
xdoc = XDocument.Parse(myXML);
//Add new Element or appending new element in xml document at the ending
xdoc.Element("Courses").Add(new XElement("Course", "Phython"));
//Add new Element at First
xdoc.Element("Courses").AddFirst(new XElement("Course", "SQL Server"));
var result = xdoc.Element("Courses").Descendants();
foreach (XElement item in result)
{
Console.WriteLine("Course Name - " + item.Value);
}
Console.ReadKey();
}
}
}
Output:
SQL Server
PHP
JAVA
DOTNET
Phython
Example of LINQ to XML to remove or delete xml tag
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQApp
{
class Program
{
static void Main(string[] args)
{//Creating xml document text in string
string XML = @"<Courses>
<Course>DotNET</Course>
<Course>JAVA</Course>
<Course>PHP</Course>
<Course>SQL Server</Course>
</Courses>";
XDocument xdoc = new XDocument();
//Converting string type to XML Document format
xdoc = XDocument.Parse(XML);
//Querying to delete where course value matched with JAVA
xdoc.Descendants().Where(s => s.Value == "JAVA").Remove();
//Retreiving all courses from xml document
var items = xdoc.Element("Courses").Descendants();
//Printing all course names
foreach (string s in items)
{
Console.WriteLine("Course Name: " + s);
}
Console.ReadKey();
}
}
}
Example program for LINQ to XML:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQApp
{
class Program
{
static void Main(string[] args)
{
//xml data in saved in string
string myXML = @"<Students>
<StudentName>Amir</StudentName>
<StudentName>Salman</StudentName>
<StudentName>Sharukh</StudentName>
<StudentName>Hrithik</StudentName>
</Students>";
XDocument xdoc = new XDocument();
//Converting data in string to XDocument format.
xdoc = XDocument.Parse(myXML);
//Quering all elements under Students tag
var result = xdoc.Element("Students").Descendants();
//Printing Values inside all retreived nodes(Nodes inside Students node)
foreach (XElement item in result)
{
Console.WriteLine("Student Name - " + item.Value);
}
Console.ReadKey();
}
}
}
Example program to Add new xml tags in exiting xml document:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQApp
{
class Program
{
static void Main(string[] args)
{
string myXML = @"<Courses>
<Course>PHP</Course>
<Course>JAVA</Course>
<Course>DOT NET</Course>
</Courses>";
XDocument xdoc = new XDocument();
xdoc = XDocument.Parse(myXML);
//Add new Element or appending new element in xml document at the ending
xdoc.Element("Courses").Add(new XElement("Course", "Phython"));
//Add new Element at First
xdoc.Element("Courses").AddFirst(new XElement("Course", "SQL Server"));
var result = xdoc.Element("Courses").Descendants();
foreach (XElement item in result)
{
Console.WriteLine("Course Name - " + item.Value);
}
Console.ReadKey();
}
}
}
Output:
SQL Server
PHP
JAVA
DOTNET
Phython
Example of LINQ to XML to remove or delete xml tag
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQApp
{
class Program
{
static void Main(string[] args)
{//Creating xml document text in string
string XML = @"<Courses>
<Course>DotNET</Course>
<Course>JAVA</Course>
<Course>PHP</Course>
<Course>SQL Server</Course>
</Courses>";
XDocument xdoc = new XDocument();
//Converting string type to XML Document format
xdoc = XDocument.Parse(XML);
//Querying to delete where course value matched with JAVA
xdoc.Descendants().Where(s => s.Value == "JAVA").Remove();
//Retreiving all courses from xml document
var items = xdoc.Element("Courses").Descendants();
//Printing all course names
foreach (string s in items)
{
Console.WriteLine("Course Name: " + s);
}
Console.ReadKey();
}
}
}
Output
DotNET
PHP
SQL Server
PHP
SQL Server
LINQ TUTORIAL PART 3 - LINQ to OBJECTS
LINQ to Objects queries will return data of IEnumerable type.
Example of LINQ to Object with array type:
using System;
using System.Linq;
namespace LINQApp
{
class Program
{
static void Main(string[] args)
{//Fruit names are created in an array object
string[] fruits = { "Guava", "Orange", "Apple", "Mango", "Banana" };
//All items from fruits array are queried using LINQ query
var items = from f in fruits
select f;
foreach (string s in items)
{
Console.Write(s + Environment.NewLine);
}
Console.ReadKey();
}
}
}
Output:
Guava
Orange
Apple
Mango
Banana
Example of LINQ to Objects using Custom type:
using System;
using System.Collections.Generic;
using System.Linq;
namespace LINQApp
{
//Type with two properties Name and Password
class StudentLogin
{
public string StudentName { get; set; }
public string StudentPassword { get; set; }
}
class Program
{
static void Main(string[] args)
{
//Student Object of List with StudentLogin type
List<StudentLogin> Student = new List<StudentLogin>();
//Adding 3 items to Student object
Student.Add(new StudentLogin { StudentName="Sudhir",StudentPassword="1234"});
Student.Add(new StudentLogin { StudentName = "Ram", StudentPassword = "Ramu" });
Student.Add(new StudentLogin { StudentName = "Raj", StudentPassword = "Raju" });
//Querying all items in Student Object
var stud = from st in Student
select st;
//Printing Name and passwords retreived using LINQ query
foreach (var s in stud)
{
Console.WriteLine(s.StudentName +" , "+s.StudentPassword);
}
Console.ReadKey();
}
}
}
Example of LINQ to Object with array type:
using System;
using System.Linq;
namespace LINQApp
{
class Program
{
static void Main(string[] args)
{//Fruit names are created in an array object
string[] fruits = { "Guava", "Orange", "Apple", "Mango", "Banana" };
//All items from fruits array are queried using LINQ query
var items = from f in fruits
select f;
foreach (string s in items)
{
Console.Write(s + Environment.NewLine);
}
Console.ReadKey();
}
}
}
Output:
Guava
Orange
Apple
Mango
Banana
Example of LINQ to Objects using Custom type:
using System;
using System.Collections.Generic;
using System.Linq;
namespace LINQApp
{
//Type with two properties Name and Password
class StudentLogin
{
public string StudentName { get; set; }
public string StudentPassword { get; set; }
}
class Program
{
static void Main(string[] args)
{
//Student Object of List with StudentLogin type
List<StudentLogin> Student = new List<StudentLogin>();
//Adding 3 items to Student object
Student.Add(new StudentLogin { StudentName="Sudhir",StudentPassword="1234"});
Student.Add(new StudentLogin { StudentName = "Ram", StudentPassword = "Ramu" });
Student.Add(new StudentLogin { StudentName = "Raj", StudentPassword = "Raju" });
//Querying all items in Student Object
var stud = from st in Student
select st;
//Printing Name and passwords retreived using LINQ query
foreach (var s in stud)
{
Console.WriteLine(s.StudentName +" , "+s.StudentPassword);
}
Console.ReadKey();
}
}
}
Output:
Sudhir , 1234
Ram , Ramu
Raj , Raju
LINQ TUTORIAL PART 2 - LINQ to SQL
LINQ to SQL is also known as DLINQ. It is used to query data in SQL server database by using LINQ expressions.
Example:
Right click on data connections in server explorer.
Add connection -> enter servername, authentication, database name.
Test connection and click on ok.
Right click on your project in solution explorer
Click on Add item -> Add linq to Sql class(.dbml file) to your project
Open tables in new data connection added in server explorer and drag them to design view of linq to sql class.
Program in console application to display data from database:
using System;
using System.Linq;
namespace ConsoleApplication8
{
class Program
{
static void Main(string[] args)
{
//You can see this context name in .dbml file in designer.cs class
DataClasses1DataContext db = new DataClasses1DataContext("Data Source=.;Initial Catalog=stdntdb;Integrated Security=True");
stdnttable newStdnt = new stdnttable();
//Select command to retreive all rows from the table
var q =
from a in db.GetTable<stdnttable>()
select a;
//printing all rows of data
foreach (var s in q)
{
Console.WriteLine("username: " + s.username + ", Password: " + s.pwrd);
}
//Select command with where condition to display names starting with sa
Console.WriteLine("");
var q1 =
from a in db.GetTable<stdnttable>() where a.username.StartsWith("sa")
select a;
//printing all rows of data
foreach (var s in q1)
{
Console.WriteLine("username: " + s.username + ", Password: " + s.pwrd);
}
Console.ReadKey();
}
}
}
Example:
Right click on data connections in server explorer.
Add connection -> enter servername, authentication, database name.
Test connection and click on ok.
Right click on your project in solution explorer
Click on Add item -> Add linq to Sql class(.dbml file) to your project
Open tables in new data connection added in server explorer and drag them to design view of linq to sql class.
Program in console application to display data from database:
using System;
using System.Linq;
namespace ConsoleApplication8
{
class Program
{
static void Main(string[] args)
{
//You can see this context name in .dbml file in designer.cs class
DataClasses1DataContext db = new DataClasses1DataContext("Data Source=.;Initial Catalog=stdntdb;Integrated Security=True");
stdnttable newStdnt = new stdnttable();
//Select command to retreive all rows from the table
var q =
from a in db.GetTable<stdnttable>()
select a;
//printing all rows of data
foreach (var s in q)
{
Console.WriteLine("username: " + s.username + ", Password: " + s.pwrd);
}
//Select command with where condition to display names starting with sa
Console.WriteLine("");
var q1 =
from a in db.GetTable<stdnttable>() where a.username.StartsWith("sa")
select a;
//printing all rows of data
foreach (var s in q1)
{
Console.WriteLine("username: " + s.username + ", Password: " + s.pwrd);
}
Console.ReadKey();
}
}
}
LINQ TUTORIAL PART 1 - INTRODUCTION
LINQ stands for language integrated query.
It is a simple way to query SQL data, XML data or other C# objects.
It is written along with C# code hence it is known as language integrated query.
LINQ provides intellisense which helps in accurate and easy querying.
Debugging is available for writing complex logics easily.
System.Linq is the namespace used for LINQ.
Types of LINQ:
LINQ to Objects
LINQ to XML
LINQ to DataSeet
LINQ to SQL
LINQ to Entities
Example to query data from C#.NET array in console application:
using System;
using System.Linq;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
//Created array with 5 numbers in it
int[] array = new int[5] { 3, 7, 1, 7, 5 };
//Var i contains data retreived using linq query
//values greater than 5 are retreived
var i = from s in array where s > 5 select s;
//Retreived data is printed using for each loop
foreach (int j in i)
{
Console.WriteLine(j);
}
Console.ReadKey();
}
}
}
Output:
7
7
It is a simple way to query SQL data, XML data or other C# objects.
It is written along with C# code hence it is known as language integrated query.
LINQ provides intellisense which helps in accurate and easy querying.
Debugging is available for writing complex logics easily.
System.Linq is the namespace used for LINQ.
Types of LINQ:
LINQ to Objects
LINQ to XML
LINQ to DataSeet
LINQ to SQL
LINQ to Entities
Example to query data from C#.NET array in console application:
using System;
using System.Linq;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
//Created array with 5 numbers in it
int[] array = new int[5] { 3, 7, 1, 7, 5 };
//Var i contains data retreived using linq query
//values greater than 5 are retreived
var i = from s in array where s > 5 select s;
//Retreived data is printed using for each loop
foreach (int j in i)
{
Console.WriteLine(j);
}
Console.ReadKey();
}
}
}
Output:
7
7